UISearchBar
创建
UISearchBar *searchBar = [[UISearchBar alloc] initWithFrame:frame];
样式(区别如图所示)
@property (nonatomic) UISearchBarStyle searchBarStyle
searchBar.searchBarStyle = UISearchBarStyleDefault;
UISearchBarStyleMinimal;
UISearchBarStyleProminent
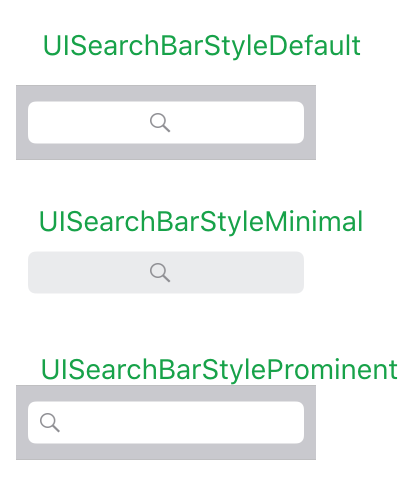
搜索框样式(区别如图所示)
@property(nonatomic) UIBarStyle barStyle
searchBar.barStyle = UIBarStyleDefault; = 0,
UIBarStyleBlack = 1,
UIBarStyleBlackOpaque = 1,
UIBarStyleBlackTranslucent = 2,
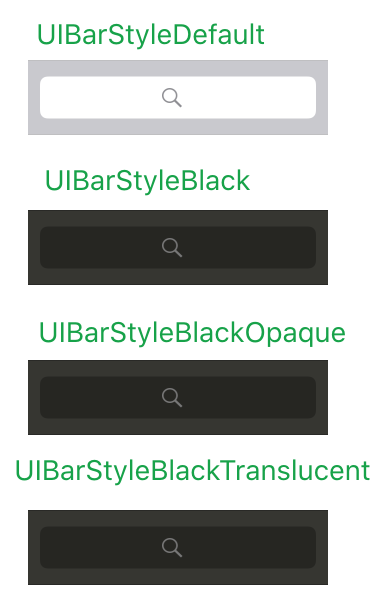
text
@property(nullable,nonatomic,copy) NSString *text;
searchBar.text = @"UISearchBar";
placeholder
@property(nullable,nonatomic,copy) NSString *placeholder;
searchBar.placeholder = @"Placeholder";
textField和textField的输入框的偏移
searchBar.searchFieldBackgroundPositionAdjustment = UIOffsetMake(<#CGFloat horizontal#>, <#CGFloat vertical#>);
searchBar.searchTextPositionAdjustment = UIOffsetMake(<#CGFloat horizontal#>, <#CGFloat vertical#>);
UIOffsetMake(<#CGFloat horizontal#>, <#CGFloat vertical#>); // 负左正右,负上正下
辅助按钮
@property(nonatomic) BOOL showsBookmarkButton
@property(nonatomic) BOOL showsCancelButton
@property(nonatomic) BOOL showsSearchResultsButton
@property(nonatomic, getter=isSearchResultsButtonSelected) BOOL searchResultsButtonSelected
- (void)searchBarBookmarkButtonClicked:(UISearchBar *)searchBar;
- (void)searchBarCancelButtonClicked:(UISearchBar *)searchBar;
- (void)searchBarResultsListButtonClicked:(UISearchBar *)searchBar;
- (void)searchBarSearchButtonClicked:(UISearchBar *)searchBar;
tintColor && barTintColor
@property(null_resettable, nonatomic,strong) UIColor *tintColor;
@property(nullable, nonatomic,strong) UIColor *barTintColor
translucent
@property(nonatomic,assign,getter=isTranslucent) BOOL translucent
附属选项视图
searchBar.showsScopeBar = YES;
searchBar.scopeButtonTitles = [NSMutableArray arrayWithObjects:@"One", @"Two", @"Three",nil];
searchBar.selectedScopeButtonIndex = 1;
修改默认小图标
- (void)setImage:(nullable UIImage *)iconImage forSearchBarIcon:(UISearchBarIcon)icon state:(UIControlState)state {};
typedef NS_ENUM(NSInteger, UISearchBarIcon) {
UISearchBarIconSearch,
UISearchBarIconClear ,
UISearchBarIconBookmark ,
UISearchBarIconResultsList,
};
键盘的附属视图如图:
@property (nullable, nonatomic, readwrite, strong) UIView *inputAccessoryView;
UIView *redView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 0, 30)];
redView.backgroundColor = [UIColor redColor];
searchBar.inputAccessoryView = redView;

搜索框的背景如图:
@property(nullable, nonatomic,strong) UIImage *backgroundImage;
UIImage *redImage = [UIImage imageNamed:@"1.png"];
searchBar.backgroundImage = redImage;

附属选项视图如图:
@property(nullable, nonatomic,strong) UIImage *scopeBarBackgroundImage;
UIImage *image = [UIImage imageNamed:@"1.png"];
searchBar.scopeBarBackgroundImage = image;

UISearchBarDelegate
- (BOOL)searchBarShouldBeginEditing:(UISearchBar *)searchBar;
- (void)searchBarTextDidBeginEditing:(UISearchBar *)searchBar;
- (BOOL)searchBarShouldEndEditing:(UISearchBar *)searchBar;
- (void)searchBarTextDidEndEditing:(UISearchBar *)searchBar;
- (void)searchBar:(UISearchBar *)searchBar textDidChange:(NSString *)searchText;
- (BOOL)searchBar:(UISearchBar *)searchBar shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text NS_AVAILABLE_IOS(3_0);
- (void)searchBarSearchButtonClicked:(UISearchBar *)searchBar;
- (void)searchBarBookmarkButtonClicked:(UISearchBar *)searchBar __TVOS_PROHIBITED;
- (void)searchBarCancelButtonClicked:(UISearchBar *)searchBar __TVOS_PROHIBITED;
- (void)searchBarResultsListButtonClicked:(UISearchBar *)searchBar NS_AVAILABLE_IOS(3_2) __TVOS_PROHIBITED;
- (void)searchBar:(UISearchBar *)searchBar selectedScopeButtonIndexDidChange:(NSInteger)selectedScope NS_AVAILABLE_IOS(3_0);
获取到search.textField(UITextField细节设置查看笔记1.09)
UITextField *searchField = [_searchBar valueForKey:@"_searchField"];